برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه — راهنمای کاربردی
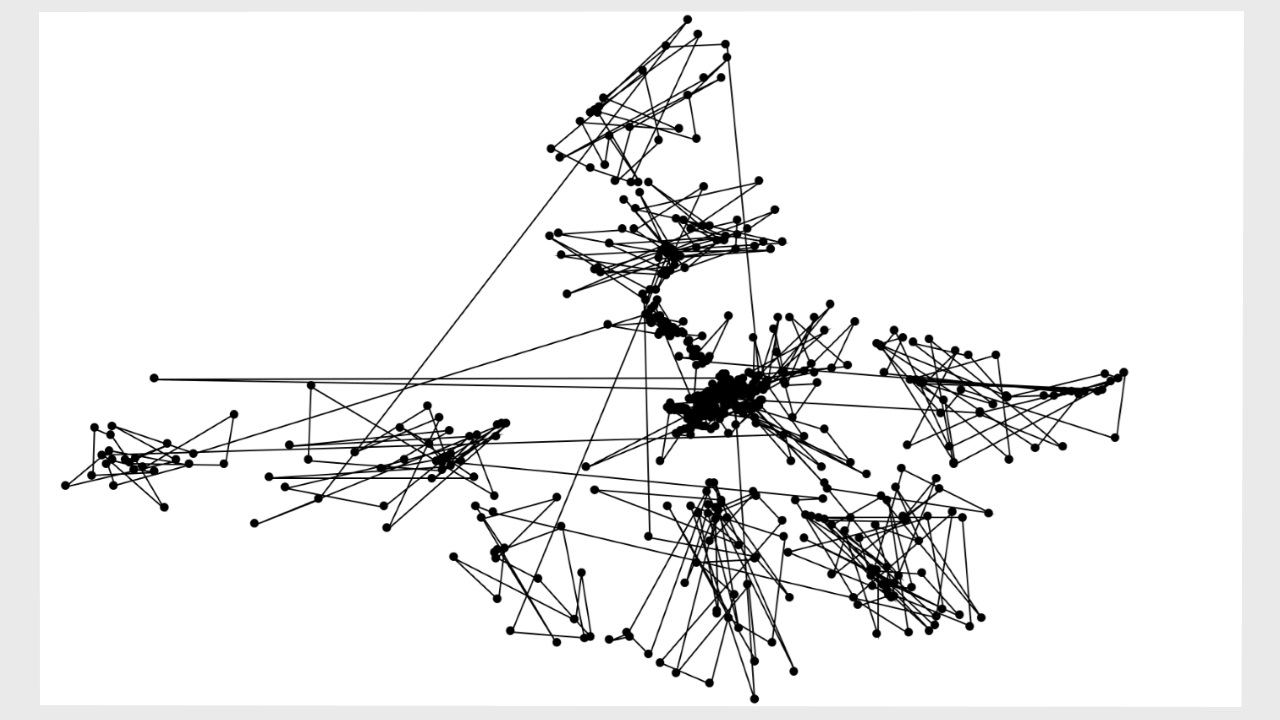
در این مطلب، روش نوشتن برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه مورد بررسی قرار گرفته و پیادهسازی آن در زبانهای برنامهنویسی گوناگون شامل ++C، «جاوا» (Java)، «پایتون» (Python)، «سیشارپ» #C و PHP انجام شده است.
سه نقطه در مختصاتهای x-y داده شده است. هدف نوشتن برنامهای است که تعداد پارهخطهای افقی یا عمودی گذر کننده از این سه نقطه را محاسبه کند. پاره خطها میتوانند به صورت عمودی یا افقی با محور مختصات تراز شوند. مثالهای زیر برای درک بهتر موضوع، قابل توجه هستند.
مثال:
Input : A = {-1, -1}, B = {-1, 3}, C = {4, 3} Output : 2
بسط مثال بالا: دو پاره خط در این پلیلاین وجود دارند.
Input :A = {1, 1}, B = {2, 3} C = {3, 2} Output : 3
در صورتی که همه نقاط روی محورهای x و y قرار داشته باشند، نتیجه برابر با یک است. در صورتی که نقاط بتوانند شکل L را شکل دهند، نتیجه برابر با ۲ است. شکل L در صورتی شکل میگیرد که هر یک از سه نقطه به عنوان یک نقطه اتصال قابل استفاده باشند.
برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه در ++C
1// CPP program to find number of horizontal (or vertical
2// line segments needed to connect three points.
3#include <iostream>
4using namespace std;
5
6// Function to check if the third point forms a
7// rectangle with other two points at corners
8bool isBetween(int a, int b, int c)
9{
10 return min(a, b) <= c && c <= max(a, b);
11}
12
13// Returns true if point k can be used as a joining
14// point to connect using two line segments
15bool canJoin(int x[], int y[], int i, int j, int k)
16{
17 // Check for the valid polyline with two segments
18 return (x[k] == x[i] || x[k] == x[j]) &&
19 isBetween(y[i], y[j], y[k]) ||
20 (y[k] == y[i] || y[k] == y[j]) &&
21 isBetween(x[i], x[j], x[k]);
22}
23
24int countLineSegments(int x[], int y[])
25{
26 // Check whether the X-coordinates or
27 // Y-cocordinates are same.
28 if ((x[0] == x[1] && x[1] == x[2]) ||
29 (y[0] == y[1] && y[1] == y[2]))
30 return 1;
31
32 // Iterate over all pairs to check for two
33 // line segments
34 else if (canJoin(x, y, 0, 1, 2) ||
35 canJoin(x, y, 0, 2, 1) ||
36 canJoin(x, y, 1, 2, 0))
37 return 2;
38
39 // Otherwise answer is three.
40 else
41 return 3;
42}
43
44// Driver code
45int main()
46{
47 int x[3], y[3];
48 x[0] = -1; y[0] = -1;
49 x[1] = -1; y[1] = 3;
50 x[2] = 4; y[2] = 3;
51 cout << countLineSegments(x, y);
52 return 0;
53}
برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه در جاوا
1// Java program to find number of horizontal
2// (or vertical line segments needed to
3// connect three points.
4import java.io.*;
5
6class GFG {
7
8// Function to check if the third
9// point forms a rectangle with
10// other two points at corners
11static boolean isBetween(int a, int b, int c)
12{
13 return (Math.min(a, b) <= c &&
14 c <= Math.max(a, b));
15}
16
17// Returns true if point k can be
18// used as a joining point to connect
19// using two line segments
20static boolean canJoin(int x[], int y[],
21 int i, int j, int k)
22{
23 // Check for the valid polyline
24 // with two segments
25 return (x[k] == x[i] || x[k] == x[j]) &&
26 isBetween(y[i], y[j], y[k]) ||
27 (y[k] == y[i] || y[k] == y[j]) &&
28 isBetween(x[i], x[j], x[k]);
29}
30
31static int countLineSegments(int x[], int y[])
32{
33 // Check whether the X-coordinates or
34 // Y-cocordinates are same.
35 if ((x[0] == x[1] && x[1] == x[2]) ||
36 (y[0] == y[1] && y[1] == y[2]))
37 return 1;
38
39 // Iterate over all pairs to check for two
40 // line segments
41 else if (canJoin(x, y, 0, 1, 2) ||
42 canJoin(x, y, 0, 2, 1) ||
43 canJoin(x, y, 1, 2, 0))
44 return 2;
45
46 // Otherwise answer is three.
47 else
48 return 3;
49}
50
51// Driver code
52public static void main (String[] args) {
53
54 int x[]=new int[3], y[]=new int[3];
55
56 x[0] = -1; y[0] = -1;
57 x[1] = -1; y[1] = 3;
58 x[2] = 4; y[2] = 3;
59
60 System.out.println(countLineSegments(x, y));
61 }
62
63
64}
65
66// This code is contributed by vt_m
برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه در پایتون ۳
1# Python program to find number
2# of horizontal (or vertical
3# line segments needed to
4# connect three points.
5
6import math
7
8# Function to check if the
9# third point forms a
10# rectangle with other
11# two points at corners
12def isBetween(a, b, c) :
13
14 return min(a, b) <= c and c <= max(a, b)
15
16
17# Returns true if point k
18# can be used as a joining
19# point to connect using
20# two line segments
21def canJoin( x, y, i, j, k) :
22
23 # Check for the valid polyline
24 # with two segments
25 return (x[k] == x[i] or x[k] == x[j]) and isBetween(y[i], y[j], y[k]) or (y[k] == y[i] or y[k] == y[j]) and isBetween(x[i], x[j], x[k])
26
27
28def countLineSegments( x, y):
29
30 # Check whether the X-coordinates or
31 # Y-cocordinates are same.
32 if ((x[0] == x[1] and x[1] == x[2]) or
33 (y[0] == y[1] and y[1] == y[2])):
34 return 1
35
36 # Iterate over all pairs to check for two
37 # line segments
38 elif (canJoin(x, y, 0, 1, 2) or
39 canJoin(x, y, 0, 2, 1) or
40 canJoin(x, y, 1, 2, 0)):
41 return 2
42
43 # Otherwise answer is three.
44 else:
45 return 3
46#driver code
47x= [-1,-1, 4]
48y= [-1, 3, 3]
49
50print(countLineSegments(x, y))
51
52# This code is contributed by Gitanjali.
برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه در #C
1// C# program to find number of horizontal
2// (or vertical) line segments needed to
3// connect three points.
4using System;
5
6class GFG {
7
8 // Function to check if the third
9 // point forms a rectangle with
10 // other two points at corners
11 static bool isBetween(int a, int b, int c)
12 {
13 return (Math.Min(a, b) <= c &&
14 c <= Math.Max(a, b));
15 }
16
17 // Returns true if point k can be
18 // used as a joining point to connect
19 // using two line segments
20 static bool canJoin(int[] x, int[] y,
21 int i, int j, int k)
22 {
23
24 // Check for the valid polyline
25 // with two segments
26 return (x[k] == x[i] || x[k] == x[j])
27 && isBetween(y[i], y[j], y[k])
28 || (y[k] == y[i] || y[k] == y[j])
29 && isBetween(x[i], x[j], x[k]);
30 }
31
32 static int countLineSegments(int[] x, int[] y)
33 {
34
35 // Check whether the X-coordinates or
36 // Y-cocordinates are same.
37 if ((x[0] == x[1] && x[1] == x[2]) ||
38 (y[0] == y[1] && y[1] == y[2]))
39 return 1;
40
41 // Iterate over all pairs to check for two
42 // line segments
43 else if (canJoin(x, y, 0, 1, 2)
44 || canJoin(x, y, 0, 2, 1)
45 || canJoin(x, y, 1, 2, 0))
46 return 2;
47
48 // Otherwise answer is three.
49 else
50 return 3;
51 }
52
53 // Driver code
54 public static void Main()
55 {
56
57 int[] x = new int[3];
58 int[] y = new int[3];
59
60 x[0] = -1;
61 y[0] = -1;
62 x[1] = -1;
63 y[1] = 3;
64 x[2] = 4;
65 y[2] = 3;
66
67 Console.WriteLine(countLineSegments(x, y));
68 }
69}
70
71// This code is contributed by vt_m.
برنامه محاسبه تعداد پارهخط های متصل کننده سه نقطه در PHP
1<?php
2// PHP program to find number
3// of horizontal (or vertical
4// line segments needed to
5// connect three points.
6
7
8// Function to check if the
9// third point forms a
10// rectangle with other
11// two points at corners
12function isBetween( $a, $b, $c)
13{
14 return min($a, $b) <= $c and
15 $c <= max($a, $b);
16}
17
18// Returns true if point k
19// can be used as a joining
20// point to connect using
21// two line segments
22function canJoin($x, $y, $i, $j, $k)
23{
24 // Check for the valid
25 // polyline with two segments
26 return ($x[$k] == $x[$i] or
27 $x[$k] == $x[$j]) and
28 isBetween($y[$i], $y[$j], $y[$k]) or
29 ($y[$k] == $y[$i] or
30 $y[$k] == $y[$j]) and
31 isBetween($x[$i], $x[$j], $x[$k]);
32}
33
34function countLineSegments( $x, $y)
35{
36 // Check whether the X-coordinates
37 // or Y-cocordinates are same.
38 if (($x[0] == $x[1] and $x[1] == $x[2]) or
39 ($y[0] == $y[1] and $y[1] == $y[2]))
40 return 1;
41
42 // Iterate over all pairs to
43 // check for two line segments
44 else if (canJoin($x, $y, 0, 1, 2) or
45 canJoin($x, $y, 0, 2, 1) ||
46 canJoin($x, $y, 1, 2, 0))
47 return 2;
48
49 // Otherwise answer is three.
50 else
51 return 3;
52}
53
54// Driver code
55$x = array();
56$y = array();
57$x[0] = -1; $y[0] = -1;
58$x[1] = -1; $y[1] = 3;
59$x[2] = 4; $y[2] = 3;
60echo countLineSegments($x, $y);
61
62// This code is contributed by anuj_67.
63?>
اگر نوشته بالا برای شما مفید بوده است، آموزشهای زیر نیز به شما پیشنهاد میشوند:
- مجموعه آموزشهای برنامهنویسی
- آموزش برنامهنویسی C++
- مجموعه آموزشهای ریاضیات
- یافتن دور همیلتونی با الگوریتم پس گرد — به زبان ساده
- الگوریتم بازی مار و پله همراه با کد — به زبان ساده
- حل مساله n وزیر با الگوریتم پسگرد (Backtracking) — به زبان ساده
- الگوریتم جست و جوی دودویی در جاوا اسکریپت — به زبان ساده
^^