برنامه تشخیص عدد توان دو — از صفر تا صد
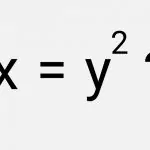
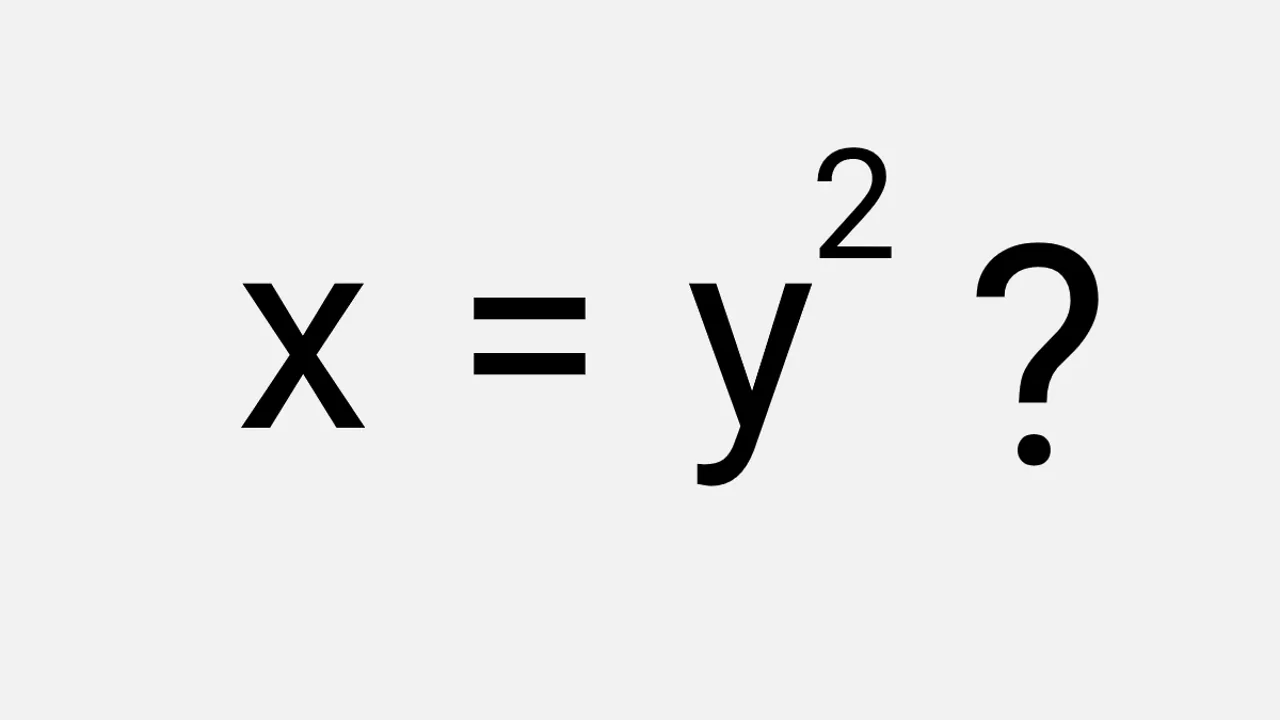
در این مطلب، روش نوشتن برنامه تشخیص عدد توان دو بیان شده است. یک عدد صحیح مثبت داده شده است. هدف، نوشتن تابعی است که تشخیص دهد آیا این عدد، یک عدد به توان ۲ رسیده است یا خیر. مثال زیر در این راستا قابل توجه است.
Input : n = 4 Output : Yes 22 = 4 Input : n = 7 Output : No Input : n = 32 Output : Yes 25 = 32
راهکار اول برای نوشتن برنامه تشخیص عدد توان دو
یک روش ساده برای انجام این کار آن است که لوگ عدد در مبنای ۲ گرفته شود و اگر یک عدد صحیح حاصل شد، بدین معنا است که عدد به توان ۲ رسیده است.
برنامه تشخیص عدد توان ۲ در ++C
1// C++ Program to find whether a
2// no is power of two
3#include<bits/stdc++.h>
4using namespace std;
5
6// Function to check if x is power of 2
7bool isPowerOfTwo(int n)
8{
9 if(n==0)
10 return false;
11
12 return (ceil(log2(n)) == floor(log2(n)));
13}
14
15// Driver program
16int main()
17{
18 isPowerOfTwo(31)? cout<<"Yes"<<endl: cout<<"No"<<endl;
19 isPowerOfTwo(64)? cout<<"Yes"<<endl: cout<<"No"<<endl;
20
21 return 0;
22}
23
24// This code is contributed by Surendra_Gangwar
برنامه تشخیص عدد توان ۲ در C
1// C Program to find whether a
2// no is power of two
3#include<stdio.h>
4#include<stdbool.h>
5#include<math.h>
6
7/* Function to check if x is power of 2*/
8bool isPowerOfTwo(int n)
9{
10 if(n==0)
11 return false;
12
13 return (ceil(log2(n)) == floor(log2(n)));
14}
15
16// Driver program
17int main()
18{
19 isPowerOfTwo(31)? printf("Yes\n"): printf("No\n");
20 isPowerOfTwo(64)? printf("Yes\n"): printf("No\n");
21 return 0;
22}
23
24// This code is contributed by bibhudhendra
برنامه تشخیص عدد توان ۲ در جاوا
1// Java Program to find whether a
2// no is power of two
3class GFG
4{
5/* Function to check if x is power of 2*/
6static boolean isPowerOfTwo(int n)
7{
8 if(n==0)
9 return false;
10
11return (int)(Math.ceil((Math.log(n) / Math.log(2)))) ==
12 (int)(Math.floor(((Math.log(n) / Math.log(2)))));
13}
14
15// Driver Code
16public static void main(String[] args)
17{
18 if(isPowerOfTwo(31))
19 System.out.println("Yes");
20 else
21 System.out.println("No");
22
23 if(isPowerOfTwo(64))
24 System.out.println("Yes");
25 else
26 System.out.println("No");
27}
28}
29
30// This code is contributed by mits
برنامه تشخیص عدد توان دو در پایتون ۳
1# Python3 Program to find
2# whether a no is
3# power of two
4import math
5
6# Function to check
7# Log base 2
8def Log2(x):
9 if x == 0:
10 return false;
11
12 return (math.log10(x) /
13 math.log10(2));
14
15# Function to check
16# if x is power of 2
17def isPowerOfTwo(n):
18 return (math.ceil(Log2(n)) ==
19 math.floor(Log2(n)));
20
21# Driver Code
22if(isPowerOfTwo(31)):
23 print("Yes");
24else:
25 print("No");
26
27if(isPowerOfTwo(64)):
28 print("Yes");
29else:
30 print("No");
31
32# This code is contributed
33# by mits
برنامه تشخیص عدد توان دو در #C
1// C# Program to find whether
2// a no is power of two
3using System;
4
5class GFG
6{
7
8/* Function to check if
9 x is power of 2*/
10static bool isPowerOfTwo(int n)
11{
12
13 if(n==0)
14 return false;
15
16 return (int)(Math.Ceiling((Math.Log(n) /
17 Math.Log(2)))) ==
18 (int)(Math.Floor(((Math.Log(n) /
19 Math.Log(2)))));
20}
21
22// Driver Code
23public static void Main()
24{
25 if(isPowerOfTwo(31))
26 Console.WriteLine("Yes");
27 else
28 Console.WriteLine("No");
29
30 if(isPowerOfTwo(64))
31 Console.WriteLine("Yes");
32 else
33 Console.WriteLine("No");
34}
35}
36
37// This code is contributed
38// by Akanksha Rai(Abby_akku)
برنامه تشخیص عدد توان دو در PHP
1<?php
2// PHP Program to find
3// whether a no is
4// power of two
5
6// Function to check
7// Log base 2
8function Log2($x)
9{
10 return (log10($x) /
11 log10(2));
12}
13
14
15// Function to check
16// if x is power of 2
17function isPowerOfTwo($n)
18{
19 return (ceil(Log2($n)) ==
20 floor(Log2($n)));
21}
22
23// Driver Code
24if(isPowerOfTwo(31))
25echo "Yes\n";
26else
27echo "No\n";
28
29if(isPowerOfTwo(64))
30echo "Yes\n";
31else
32echo "No\n";
33
34// This code is contributed
35// by Sam007
36?>
خروجی قطعه کد بالا به صورت زیر است.
No Yes
راهکار دوم برای نوشتن برنامه تشخیص عدد توان دو
راهکار دیگر برای نوشتن برنامه تشخیص عدد توان، تقسیم کردن عدد به دو، به صورت مکرر است. یعنی، به صورت تکرار شونده انجام میشود. در هر تکرار، اگر n%2 (باقیمانده تقسیم عدد بر دو) عددی غیر صفر و n برابر با ۱ نباشد، n عددی به توان ۲ نیست. اگر n برابر با ۱ شود، عددی به توان ۲ است.
برنامه تشخیص عدد توان دو در ++C
1#include <bits/stdc++.h>
2using namespace std;
3
4/* Function to check if x is power of 2*/
5bool isPowerOfTwo(int n)
6{
7 if (n == 0)
8 return 0;
9 while (n != 1)
10 {
11 if (n%2 != 0)
12 return 0;
13 n = n/2;
14 }
15 return 1;
16}
17
18/*Driver code*/
19int main()
20{
21 isPowerOfTwo(31)? cout<<"Yes\n": cout<<"No\n";
22 isPowerOfTwo(64)? cout<<"Yes\n": cout<<"No\n";
23 return 0;
24}
25
26// This code is contributed by rathbhupendra
برنامه تشخیص عدد توان دو در C
1#include<stdio.h>
2#include<stdbool.h>
3
4/* Function to check if x is power of 2*/
5bool isPowerOfTwo(int n)
6{
7 if (n == 0)
8 return 0;
9 while (n != 1)
10 {
11 if (n%2 != 0)
12 return 0;
13 n = n/2;
14 }
15 return 1;
16}
17
18/*Driver program to test above function*/
19int main()
20{
21 isPowerOfTwo(31)? printf("Yes\n"): printf("No\n");
22 isPowerOfTwo(64)? printf("Yes\n"): printf("No\n");
23 return 0;
24}
برنامه تشخیص عدد توان دو در جاوا
1// Java program to find whether
2// a no is power of two
3import java.io.*;
4
5class GFG {
6
7 // Function to check if
8 // x is power of 2
9 static boolean isPowerOfTwo(int n)
10 {
11 if (n == 0)
12 return false;
13
14 while (n != 1)
15 {
16 if (n % 2 != 0)
17 return false;
18 n = n / 2;
19 }
20 return true;
21 }
22
23 // Driver program
24 public static void main(String args[])
25 {
26 if (isPowerOfTwo(31))
27 System.out.println("Yes");
28 else
29 System.out.println("No");
30
31 if (isPowerOfTwo(64))
32 System.out.println("Yes");
33 else
34 System.out.println("No");
35 }
36}
37
38// This code is contributed by Nikita tiwari.
برنامه تشخیص عدد توان دو در پایتون ۳
1# Python program to check if given
2# number is power of 2 or not
3
4# Function to check if x is power of 2
5def isPowerOfTwo(n):
6 if (n == 0):
7 return False
8 while (n != 1):
9 if (n % 2 != 0):
10 return False
11 n = n // 2
12
13 return True
14
15# Driver code
16if(isPowerOfTwo(31)):
17 print('Yes')
18else:
19 print('No')
20if(isPowerOfTwo(64)):
21 print('Yes')
22else:
23 print('No')
24
25# This code is contributed by Danish Raza
برنامه تشخیص عدد توان دو در #C
1// C# program to find whether
2// a no is power of two
3using System;
4
5class GFG
6{
7
8 // Function to check if
9 // x is power of 2
10 static bool isPowerOfTwo(int n)
11 {
12 if (n == 0)
13 return false;
14
15 while (n != 1) {
16 if (n % 2 != 0)
17 return false;
18
19 n = n / 2;
20 }
21 return true;
22 }
23
24 // Driver program
25 public static void Main()
26 {
27 Console.WriteLine(isPowerOfTwo(31) ? "Yes" : "No");
28 Console.WriteLine(isPowerOfTwo(64) ? "Yes" : "No");
29
30 }
31}
32
33// This code is contributed by Sam007
برنامه تشخیص عدد توان دو در PHP
1<?php
2
3// Function to check if
4// x is power of 2
5function isPowerOfTwo($n)
6{
7if ($n == 0)
8 return 0;
9while ($n != 1)
10{
11 if ($n % 2 != 0)
12 return 0;
13 $n = $n / 2;
14}
15return 1;
16}
17
18// Driver Code
19if(isPowerOfTwo(31))
20 echo "Yes\n";
21else
22 echo "No\n";
23
24if(isPowerOfTwo(64))
25 echo "Yes\n";
26else
27 echo "No\n";
28
29// This code is contributed
30// by Sam007
31?>
خروجی قطعه کد بالا به صورت زیر است.
No Yes
روشهای دیگری نیز برای حل این مساله وجود دارند. همه اعداد در توان ۲، تنها یک بیت ست هستند. بنابراین، با شمارش تعداد ست بیتها، اگر عدد ۱ حاصل شد، عدد در واقع یک عدد به توان ۲ رسیده است.
راهکار سوم برای نوشتن برنامه تشخیص عدد توان دو
در نهایت، یک روش دیگر نیز برای نوشتن برنامه تشخیص عدد توان دو در ادامه بیان شده است. اگر اعداد توان ۲ تقسیم بر ۱ شوند، همه بیتهای غیر ست پس از تنها بیت ست، ست میشوند و بیت ست شده، آنست میشود.
برای مثال، دو عدد 4 ( 100) و 16 (10000) در نظر گرفته میشود. خروجی زیر پس از کم کردن یک (۱) از عدد، حاصل میشود.
3 –> 011
15 –> 01111
بنابراین، اگر n عددی به توان ۲ است، and بیتوایز برای n و n-1، برابر با صفر خواهد بود. میتوان گفت که n توانی از ۲ است یا بر مبنای مقدار (n&(n-1 نیست. عبارت (n&(n-1 هنگامی که n برابر با صفر باشد کار میکند. برای کار با این مورد، عبارت به صورت (!(n& (n&(n-1 خواهد بود. در ادامه، پیادهسازی روش بیان شده در زبانهای برنامهنویسی گوناگون ارائه شده است.
برنامه تشخیص عدد توان دو در ++C
1#include <bits/stdc++.h>
2using namespace std;
3#define bool int
4
5/* Function to check if x is power of 2*/
6bool isPowerOfTwo (int x)
7{
8 /* First x in the below expression is for the case when x is 0 */
9 return x && (!(x&(x-1)));
10}
11
12/*Driver code*/
13int main()
14{
15 isPowerOfTwo(31)? cout<<"Yes\n": cout<<"No\n";
16 isPowerOfTwo(64)? cout<<"Yes\n": cout<<"No\n";
17 return 0;
18}
19
20// This code is contributed by rathbhupendra
برنامه تشخیص عدد توان دو در C
1#include<stdio.h>
2#define bool int
3
4/* Function to check if x is power of 2*/
5bool isPowerOfTwo (int x)
6{
7 /* First x in the below expression is for the case when x is 0 */
8 return x && (!(x&(x-1)));
9}
10
11/*Driver program to test above function*/
12int main()
13{
14 isPowerOfTwo(31)? printf("Yes\n"): printf("No\n");
15 isPowerOfTwo(64)? printf("Yes\n"): printf("No\n");
16 return 0;
17}