برنامه مرتب سازی ماتریس – راهنمای کاربردی
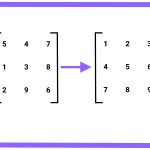
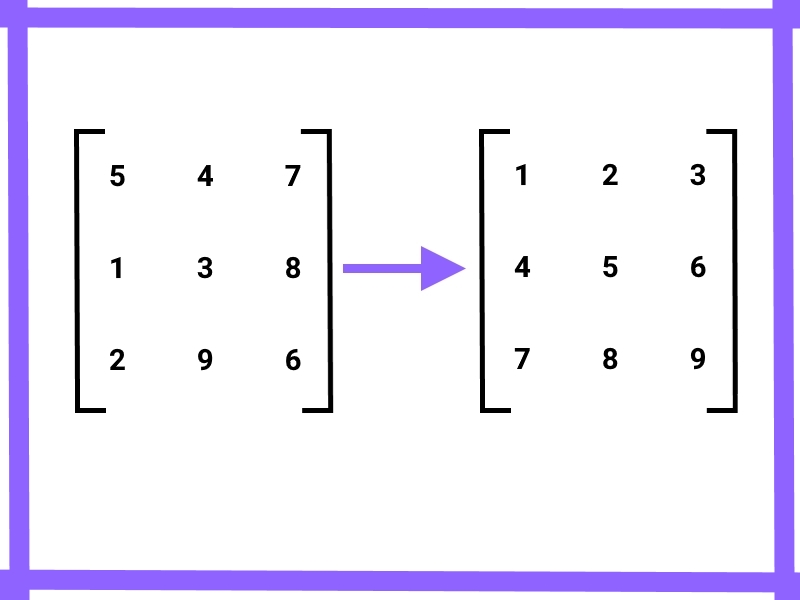
در این مطلب، روش نوشتن برنامه مرتب سازی ماتریس مورد بررسی قرار میگیرد. همچنین، پیادهسازی روش بیان شده، در زبانهای برنامهنویسی گوناگون شامل ++C، «جاوا» (Java)، «پایتون» (Python) و #C انجام شده است. برای مطالعه بیشتر راجع به ماتریسها، استفاده از دیگر مقالات مجله فرادرس پیرامون ماتریسها توصیه میشود. فرض میشود که یک ماتریس n × n داده شده است. مساله، مرتبسازی ماتریس مذکور است. در واقع، هدف نوشتن برنامهای است که ماتریس داده شده (مقادیر ماتریس) را مرتب کند. در اینجا، منظور از مرتبسازی آن است که همه عناصر در یک سطر به ترتیب صعودی باشند و برای سطر i، جایی که 1 <= i <= n-1، اولین عنصر از سطر i، بزرگتر یا مساوی آخرین عنصر از سطر i-1 است. مثال زیر در این راستا قابل توجه است.
Input : mat[][] = { {5, 4, 7}, {1, 3, 8}, {2, 9, 6} } Output : 1 2 3 4 5 6 7 8 9
برای حل مساله مذکور، ابتدا باید آرایه []temp با اندازه n2 ساخته شود. با شروع از اولین سطر، یکی یکی عناصر ماتریس داده شده در []temp کپی میشوند. سپس، []temp مرتب میشود. اکنون، عناصر []temp یکی یکی در ماتریس اصلی کپی میشوند. در ادامه، پیادهسازی روش ساده بیان شده، در زبانهای برنامهنویسی گوناگون انجام میشود. همچنین، خروجی قطعه کدها به منظور درک بهتر موضوع، در انتهای مطلب ارائه شده است.
برنامه مرتب سازی ماتریس در ++C
برنامه مرتب سازی ماتریس در جاوا
برنامه مرتب سازی ماتریس در پایتون ۳
برنامه مرتب سازی ماتریس در #C
خروجی قطعه کدهای بالا، به صورت زیر است.
Original Matrix: 5 4 7 1 3 8 2 9 6 Matrix After Sorting: 1 2 3 4 5 6 7 8 9
پیچیدگی زمانی روش ارائه شده در بالا از درجه (O(n2log2n است. پیچیدگی فضای کمکی این روش نیز از درجه (O(n2 است.
اگر نوشته بالا برای شما مفید بوده است، آموزشهای زیر نیز به شما پیشنهاد میشوند:
- مجموعه آموزشهای برنامهنویسی
- آموزش برنامهنویسی C++
- مجموعه آموزشهای ریاضیات
- یافتن دور همیلتونی با الگوریتم پس گرد — به زبان ساده
- الگوریتم بازی مار و پله همراه با کد — به زبان ساده
- حل مساله n وزیر با الگوریتم پسگرد (Backtracking) — به زبان ساده
^^