برنامه محاسبه سود و زیان – راهنمای کاربردی

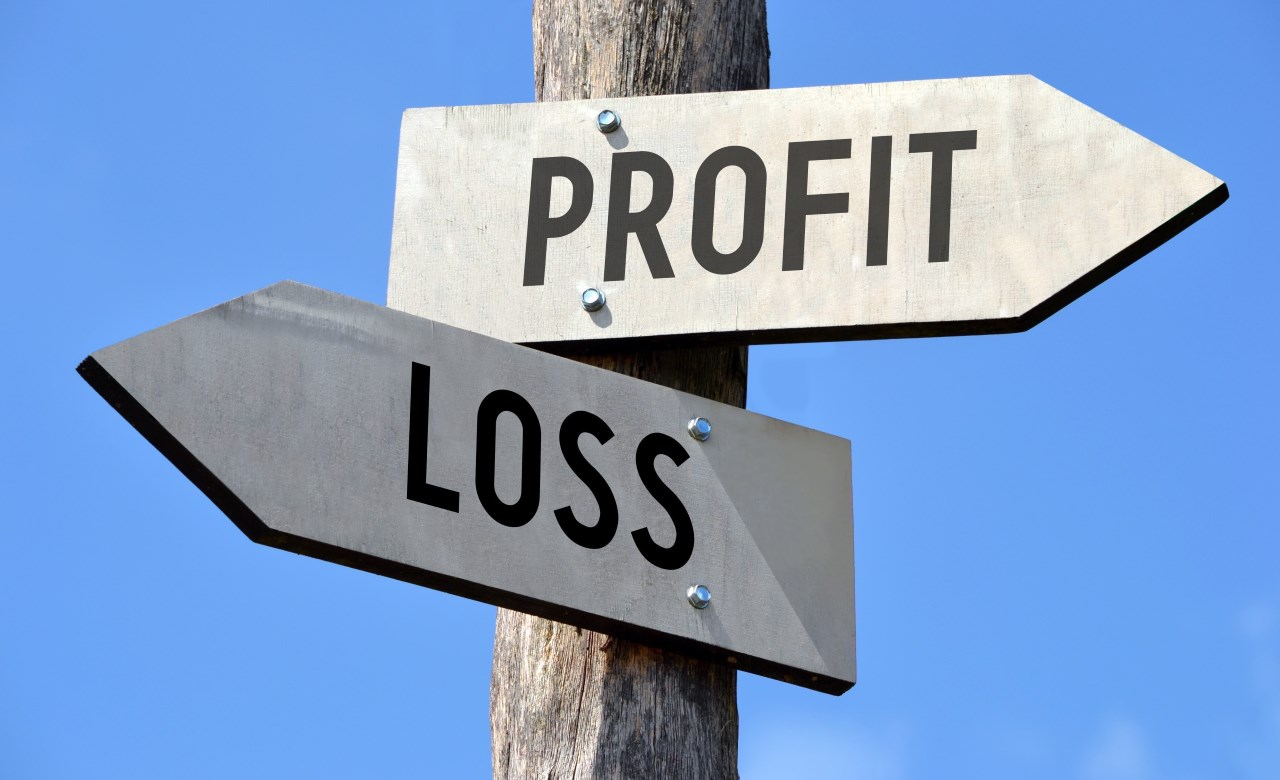
در این مطلب، روش نوشتن برنامه محاسبه سود و زیان بیان و پیادهسازی آن در زبانهای برنامهنویسی گوناگون شامل «سیپلاسپلاس» (++C)، «جاوا» (Java)، «پایتون» (Python)، «سیشارپ» (#C) و «پیاچ پی» (PHP) انجام شده است.
برنامه محاسبه سود و زیان
«قیمت خرید» (Cost Price | CP) و «قیمت فروش» (Selling Price | SP) یک محصول داده شده است.
هدف، محاسبه سود یا زیان است. مثال زیر به درک بهتر این مسئله، کمک میکند.
Input: CP = 1500, SP = 2000 Output: 500 Profit Input: CP = 3125, SP = 1125 Output: 2000 Loss
برای محاسبه سود و زیان، از رابطههای زیر استفاده میشود.
- قیمت خرید - قیمت فروش = سود
- قیمت فروش - قیمت خرید = زیان
در ادامه، پیادهسازی آنچه بیان شد، در زبانهای برنامهنویسی گوناگون انجام شده است. شایان توجه است که در برنامههای ارائه شده، فرمول سود پیادهسازی شده است. برای محاسبه زیان، میتوان کد را تغییر داد و فرمول محاسبه زیان را که در بالا نیز به آن اشاره شده است، جایگزین کرد.
برنامه محاسبه سود و زیان در ++C
1// C++ code to demonstrate Profit and Loss
2#include <iostream>
3using namespace std;
4
5// Function to calculate Profit.
6int Profit(int costPrice, int sellingPrice)
7{
8 int profit = (sellingPrice - costPrice);
9
10 return profit;
11}
12
13// Function to calculate Loss.
14int Loss(int costPrice, int sellingPrice)
15{
16 int Loss = (costPrice - sellingPrice);
17
18 return Loss;
19}
20
21// Driver Code.
22int main()
23{
24 int costPrice = 1500, sellingPrice = 2000;
25
26 if (sellingPrice == costPrice)
27 cout << "No profit nor Loss";
28
29 else if (sellingPrice > costPrice)
30 cout << Profit(costPrice, sellingPrice) << " Profit ";
31
32 else
33 cout << Loss(costPrice, sellingPrice) << " Loss ";
34
35 return 0;
36}
برنامه محاسبه سود و زیان در جاوا
1// Java code to demonstrate
2// Profit and Loss
3class GFG
4{
5// Function to calculate Profit.
6static int Profit(int costPrice,
7 int sellingPrice)
8{
9 int profit = (sellingPrice - costPrice);
10
11 return profit;
12}
13
14// Function to calculate Loss.
15static int Loss(int costPrice,
16 int sellingPrice)
17{
18 int Loss = (costPrice - sellingPrice);
19
20 return Loss;
21}
22
23// Driver Code.
24public static void main(String[] args)
25{
26 int costPrice = 1500,
27 sellingPrice = 2000;
28
29 if (sellingPrice == costPrice)
30 System.out.println("No profit nor Loss");
31
32 else if (sellingPrice > costPrice)
33 System.out.println(Profit(costPrice,
34 sellingPrice) +
35 " Profit ");
36
37 else
38 System.out.println(Loss(costPrice,
39 sellingPrice) +
40 " Loss ");
41}
42}
43
44// This code is contributed
45// by ChitraNayal
برنامه محاسبه سود و زیان در پایتون
1# Python 3 program to demonstrate
2# Profit and Loss
3
4# Function to calculate Profit.
5def Profit(costPrice, sellingPrice) :
6
7 profit = (sellingPrice - costPrice)
8
9 return profit
10
11# Function to calculate Loss.
12def Loss(costPrice, sellingPrice) :
13
14 Loss = (costPrice - sellingPrice)
15
16 return Loss
17
18# Driver code
19if __name__ == "__main__" :
20
21 costPrice, sellingPrice = 1500, 2000
22
23 if sellingPrice == costPrice :
24 print("No profit nor Loss")
25
26 elif sellingPrice > costPrice :
27 print(Profit(costPrice,
28 sellingPrice), "Profit")
29
30 else :
31 print(Loss(costPrice,
32 sellingPrice), "Loss")
33
34# This code is contributed by ANKITRAI1
برنامه محاسبه سود و زیان در #C
1// C# code to demonstrate Profit and Loss
2using System;
3class GFG
4{
5// Function to calculate Profit
6static int Profit(int costPrice,
7 int sellingPrice)
8{
9 int profit = (sellingPrice - costPrice);
10
11 return profit;
12}
13
14// Function to calculate Loss
15static int Loss(int costPrice,
16 int sellingPrice)
17{
18 int Loss = (costPrice - sellingPrice);
19
20 return Loss;
21}
22
23// Driver Code
24public static void Main()
25{
26 int costPrice = 1500,
27 sellingPrice = 2000;
28
29 if (sellingPrice == costPrice)
30 Console.Write("No profit nor Loss");
31
32 else if (sellingPrice > costPrice)
33 Console.Write(Profit(costPrice,
34 sellingPrice) + " Profit ");
35
36 else
37 Console.Write(Loss(costPrice,
38 sellingPrice) + " Loss ");
39}
40}
41
42// This code is contributed by ChitraNayal
برنامه محاسبه سود و زیان در PHP
1<?php
2// PHP code to demonstrate
3// Profit and Loss
4
5// Function to calculate Profit.
6function Profit($costPrice,
7 $sellingPrice)
8{
9 $profit = ($sellingPrice -
10 $costPrice);
11
12 return $profit;
13}
14
15// Function to calculate Loss.
16function Loss($costPrice,
17 $sellingPrice)
18{
19 $Loss = ($costPrice -
20 $sellingPrice);
21
22 return $Loss;
23}
24
25// Driver Code.
26$costPrice = 1500;
27$sellingPrice = 2000;
28
29if ($sellingPrice == $costPrice)
30 echo "No profit nor Loss";
31
32else if ($sellingPrice > $costPrice)
33 echo Profit($costPrice,
34 $sellingPrice)." Profit ";
35
36else
37 echo Loss($costPrice,
38 $sellingPrice)." Loss ";
39
40// This code is contributed
41// by ChitraNayal
42?>
خروجی قطعه کد بالا، به صورت زیر است.
500 Profit
اگر نوشته بالا برای شما مفید بوده است، آموزشهای زیر نیز به شما پیشنهاد میشوند:
- مجموعه آموزشهای برنامه نویسی
- آموزش ساختمان دادهها
- مجموعه آموزشهای ساختمان داده و طراحی الگوریتم
- رنگآمیزی گراف به روش حریصانه — به زبان ساده
- الگوریتم دایجسترا (Dijkstra) — از صفر تا صد
- الگوریتم پریم — به زبان ساده
- متن کاوی (Text Mining) — به زبان ساده
^^