عملیات در آرایه: جستجو، درج و حذف – به زبان ساده
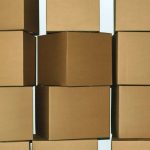
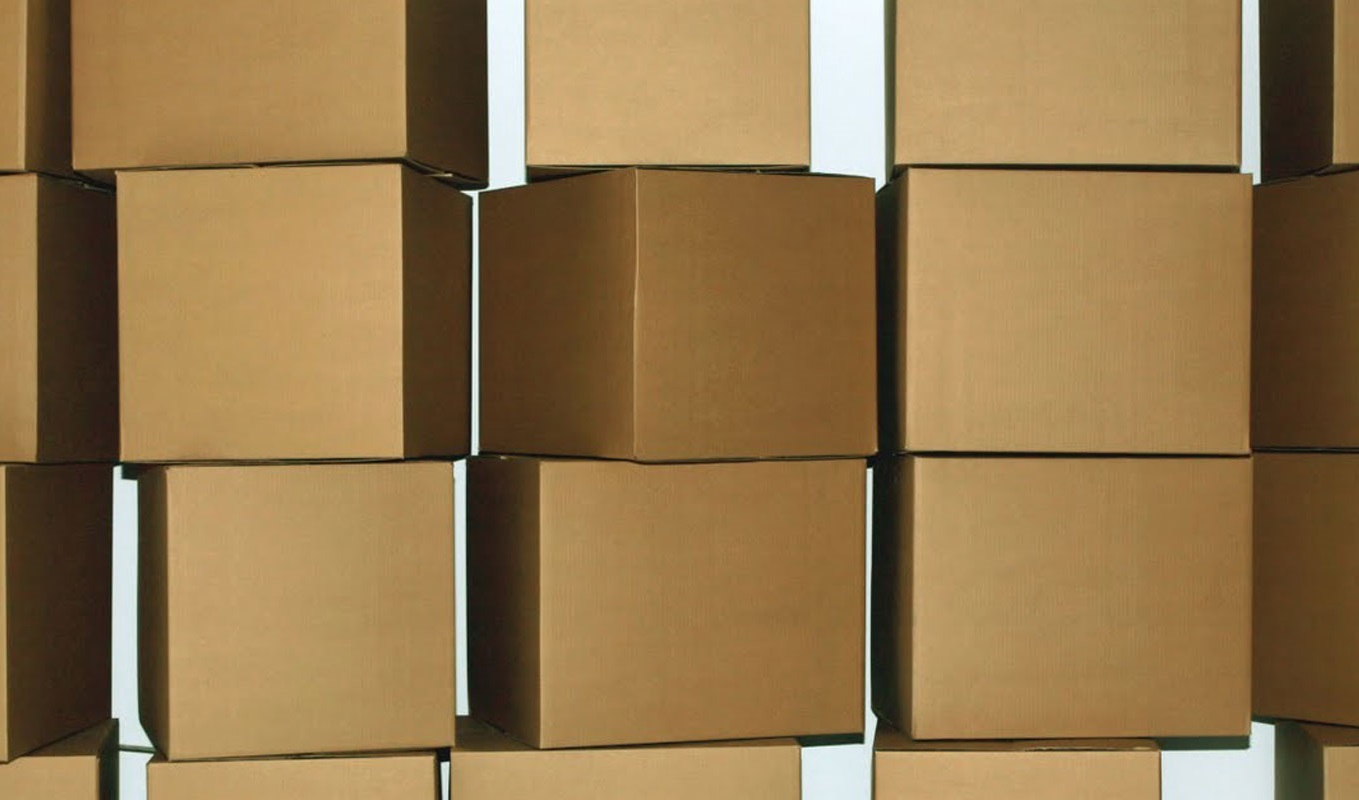
در این مطلب، انواع عملیات در آرایه شامل جستجو، درج و حذف در آرایه نامرتب مورد بررسی قرار گرفته است. همچنین، پیادهسازی این موارد در زبانهای برنامهنویسی گوناگون شامل ++C، «جاوا» (Java)، «پایتون» (Python)، «سیشارپ» (#C) و «پیاچپی» (PHP) انجام شده است.
عملیات در آرایه: جستجو در آرایه نامرتب
در یک آرایه نامرتب، عملیات جستجو را میتوان با پیمایش خطی از اولین تا آخرین عنصر انجام داد.
مثال زیر در این راستا قابل توجه است.
جستجو در آرایه نامرتب در ++C
جستجو در آرایه نامرتب در C
جستجو در آرایه نامرتب در جاوا
جستجو در آرایه نامرتب در پایتون
جستجو در آرایه نامرتب در #C
جستجو در آرایه نامرتب در PHP
خروجی قطعه کدهای بالا به صورت زیر است:
Element Found at Position: 5
پیچیدگی زمانی جستجو در آرایه نامرتب با استفاده از روشی که پیادهسازی آن در بالا انجام شده، از درجه (O(n است.
عملیات در آرایه: درج در آرایه نامرتب
در یک آرایه نامرتب، عملیات درج (Insert) در مقایسه با یک آرایه مرتب، سریعتر انجام میشود؛
زیرا نیازی به بررسی موقعیتی که عنصر باید در آن قرار بگیرد نیست.
درج در آرایه نامرتب در C
درج در آرایه نامرتب در جاوا
درج در آرایه نامرتب در پایتون
درج در آرایه نامرتب در #C
درج در آرایه نامرتب در PHP
خروجی قطعه کدهای بالا به صورت زیر است.
Before Insertion: 12 16 20 40 50 70 After Insertion: 12 16 20 40 50 70 26
پیچیدگی زمانی درج در آرایه نامرتب با استفاده از روشی که پیادهسازی آن در بالا انجام شده، از درجه (O(1 است.
عملیات در آرایه: حذف از آرایه نامرتب
در عملیات حذف، عنصری که باید حذف شود با استفاده از «جستجوی خطی» (Linear Search) مورد جستجو قرار میگیرد.
سپس، عملیات حذف با شیفت دادن عناصر انجام میشود.
حذف از آرایه نامرتب در C
حذف از آرایه نامرتب در جاوا
حذف از آرایه نامرتب در پایتون
حذف از آرایه نامرتب در #C
حذف از آرایه نامرتب در PHP
خروجی قطعه کدهای بالا، به صورت زیر است.
Array before deletion 10 50 30 40 20 Array after deletion 10 50 40 20
پیچیدگی زمانی حذف از آرایه نامرتب با استفاده از روشی که پیادهسازی آن در بالا انجام شده از درجه (O(n است.
اگر نوشته بالا برای شما مفید بوده است، آموزشهای زیر نیز به شما پیشنهاد میشوند:
- مجموعه آموزشهای برنامهنویسی
- آموزش برنامهنویسی C++
- مجموعه آموزشهای ریاضیات
- کد جمع کردن چند جملهایها — راهنمای کاربردی
- برنامه ضرب چند جملهایها — راهنمای کاربردی
^^
بابا دمتون گرم چقد جامع بود